Published 2023-06-06.
Last modified 2023-12-25.
Time to read: 5 minutes.
ruby
collection.
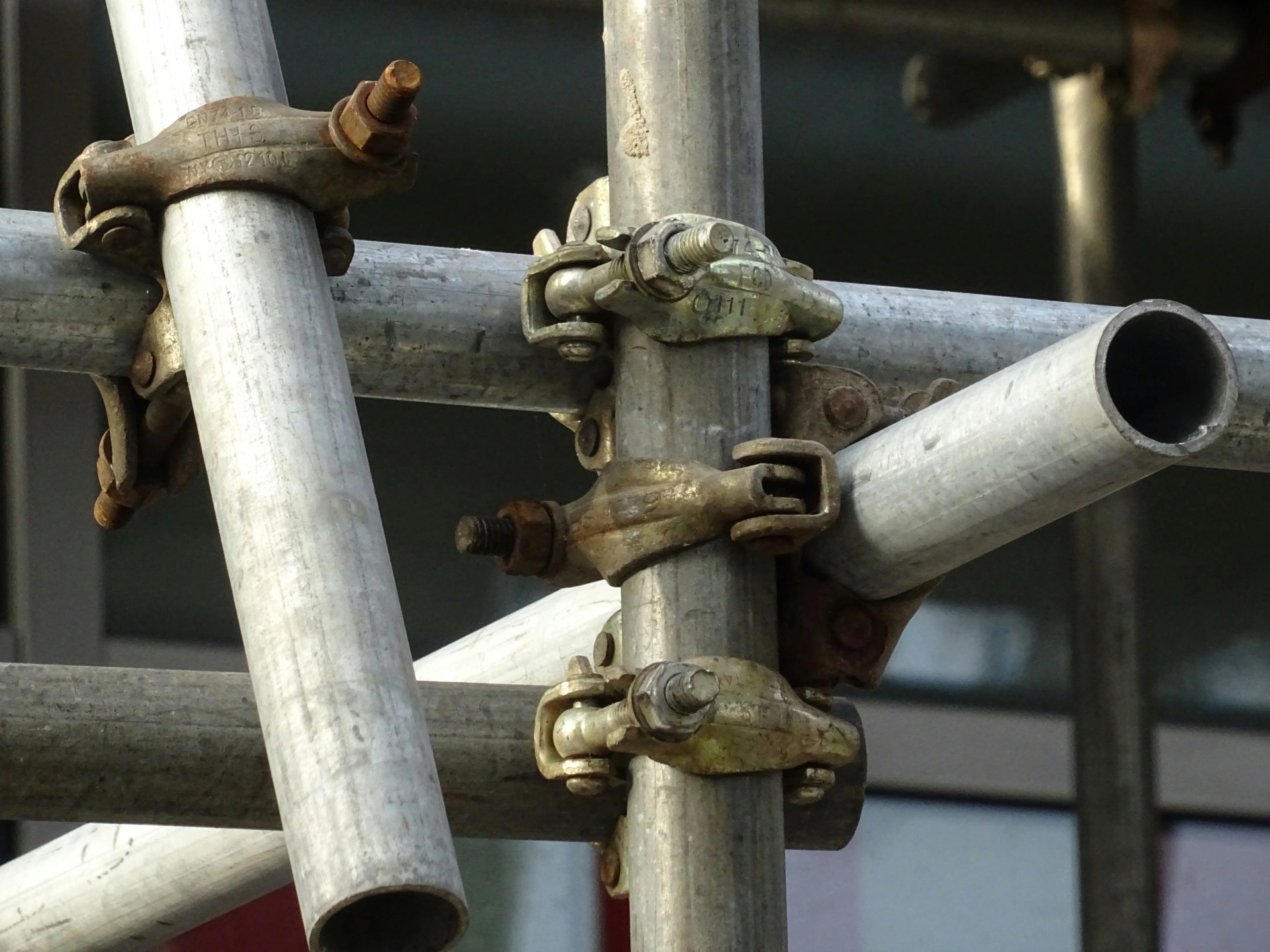
Recently, I wrote a command-line program (CLI) in Ruby. It was straightforward to write and worked well.
However, if I had used a special-purpose library for building CLIs, over the lifetime of my CLI program, the accumulated time for maintenance might have been much less. This could only be realized if the mythical library:
- Was well-designed.
- Had the necessary features.
- Continues to be well maintained.
- Had good documentation.
Unfortunately, open-source software rarely fits this description.
Writing Yet Another CLI
Next, I wanted to write another command-line program, this time a generator of various types of Ruby gems. Unlike my previous CLI project, this one had the following criteria:
- The source code needed to be easy to understand.
- The overall program needed to be flexible in how it could be used.
- The resulting software must be easy to maintain.
- Development and deployment must rely on stable technology.
I found an old Ruby gem generator, creategem
,
written by Igor Jancev.
Although the project had not been updated in seven years, it was well written.
I decided to update the project and extend it for my own use.
The project used the Thor gem.
I could have used dry-cli
or
mercenary
instead of thor
.
But I did not.
Maybe next time.
Nugem
is off to a promising start.
Check it out!
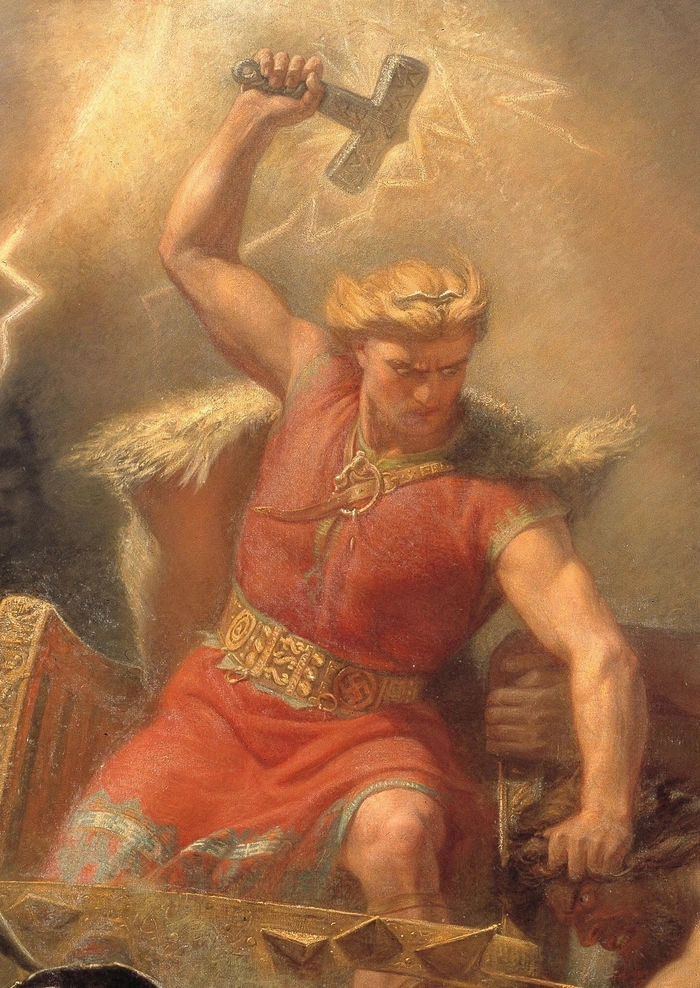
The Mighty Thor
Written by the Ruby on Rails programmers, Thor is an open-source toolkit for building powerful command-line interfaces.
This software is a classic example of programmers scratching their own itch. They were not motivated at all to help others learn how to use the program. If you want to understand how to best use Thor, you should expect to spend time reading the source code.
Despite the huge number of GitHub projects that are based on Thor,
very few of them were written by humans.
Instead, Ruby on Rails has used thor
since Rails v3 for code generation.
For example, commands like rails new
, rails console
, rails server
,
and rails routes
are implemented with thor
.
The above is not the full story.
Thor::Actions
are helpers for your Thor tasks that make typical actions, like file system interaction or
command-line user dialogue, easier.
The Actions
whose sole purpose is to interact with a user are:
ask
, indent
,
mute
, mute?
, no
,
print_in_columns
, print_table
, print_wrapped
,
say
, say_error
, set_color
,
terminal_width
, and
yes?
.
My Evaluation
Thor is:
- Well designed
- Well maintained
- Useful and powerful
-
Has no change log, and some features have changed a lot over the years.
For example, the old
option
statement was renamed some years ago tomethod_option
, but the oldoptions
variable was not renamed. I have not found any mention of that in the documentation, and it makes looking at old code confusing. - Put to shame with out-of-date and dramatically incomplete user-oriented documentation
-
Has decent comments in the source code for
Actions
and other portions of the code base. This means the RubyDoc for Thor is useful if you have time and the ability to put it all together in your mind. - Lacking in accessible examples that exercise the current API. If you have access to a working program, the task of learning the API becomes much easier.
Maybe all that is needed is for someone to write a decent book about Thor.
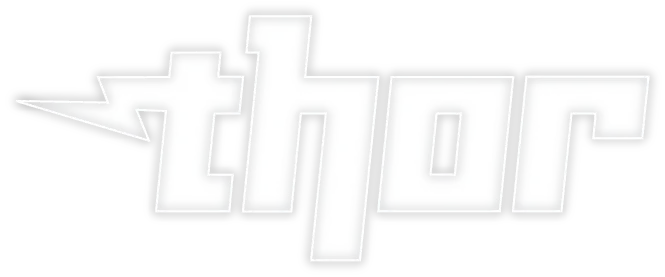
I Wrote Some Docs
Thor CLI
A command called thor
was installed when the thor
gem installed.
$ which thor /home/mslinn/.rbenv/shims/thor
$ thor help Commands: thor help [COMMAND] # Describe available commands or one specific command thor install NAME # Install an optionally named Thor file into your system commands thor installed # List the installed Thor modules and commands thor list [SEARCH] # List the available thor commands (--substring means .*SEARCH) thor uninstall NAME # Uninstall a named Thor module thor update NAME # Update a Thor file from its original location thor version # Show Thor version
Unfortunately, the help message does not state what the thor
command is for.
Google brought me to the
thor
man page,
which was weird because no such man page got installed on my Ubuntu system when I installed the thor
gem,
and apt
does not have such a package available from the default Ubuntu 23.04 PPAs.
The man page says:
Thor is a scripting framework designed for command line utilities. It is supposed to be a replacement for
rake
, sake
and rubigen
.
Really? I never would have known that from any other source. If this were true, I would expect at least some documentation. I call bullshit. Please prove me wrong.
No one needs the thor
command-line utility for any purpose,
unless they want an example of how to use thor
.
If that is something you want, the command’s source is provided in
lib/thor/runner.rb
.
Just keep in mind that this program contains a lot of use-case-specific code that probably does not match
your needs, and their use case is not expressed anywhere.
I humbly suggest that nugem
is a much better example to learn from.
BTW, rubigen
is a defunct GitHub project that vanished without a trace.
Sake
was never anything to speak of.
Never mind, thor
is a useful and valuable program,
even if its creators are woefully misguided in the documentation department.
ERB Syntax
Other than the comments in the source code, accessible via RubyDoc, I have not found any user-accessible documentation on Thor templates.
However, I realized that templates use ERB syntax after I saw error messages from erb. Since Thor has no defined dependencies that pertain to scriptlet support and ERB is built-in to Ruby, ERB syntax was apparently implemented.
Lineage
The comment
“This implementation is based in Templater actions”,
found in the Actions module source code,
suggests that this portion of the Thor source code was modified from the templater
source code
since Thor does not have the templater
gem as a dependency.
The templater
RubyDoc
seems to fit what I have noticed so far about Thor’s template capability.
This does not qualify as proper documentation for thor
,
but it should help guide future detective work.
Actions
Thor Actions
can:
- Define Thor runtime options
- Define paths for template sources
- Transform template directory contents recursively.
The code I inherited from Igor Jancev used the
directory
and template
actions.
Undocumented Run Options
The run
command is
documented
as having options :verbose
, :capture
and :with
.
Examining the
source for run,
we see the following undocumented, yet very useful, additional options:
:abort_on_failure
, :capture
, and :pretend
.
Some commands, like git-commit
, do not return zero return codes on a successful invocation.
To make them work without prematurely ending the Thor program, write:
run "git commit -aqm 'Initial commit'", abort_on_failure: false
A Few Useful Pointers
The following code shows a few handy techniques that you might find helpful:
- Exit with a non-zero return code on failure.
- Display additional information in help messages.
require 'English' require 'thor' require_relative 'bogus_cli/version' module BogusCli class CLI < Thor include Thor::Actions package_name 'BogusCli' # Return a non-zero status code on error. See https://github.com/rails/thor/issues/244 def self.exit_on_failure? true end desc('build [FILE_NAME]', 'build the future') method_option :output, type: :string, required: false, desc: 'output file', aliases: '-o' def build(file_name) # Implementation does not matter for this example end # Display help with additional context def help(command = nil, subcommand: false) say <<~END_HELP This could be a detailed overview of the CLI... END_HELP super command, subcommand end end end
Conclusion
After using Thor to write a few CLIs, I find it awkward to use.
The scant documentation makes me not want to use it.
I find that just using OptionParser
makes it quite easy to write CLIs.
References
-
Thor
GitHub project - What is Thor? – does not mention templates
- Thor Wiki
- Thor RubyDoc
- Embedded Ruby (ERB) template syntax
- Tips for writing Rails tasks with Thor instead of Rake
- Gem templates with Thor by Benjamin Fleischer
- Making a command line utility with gems and thor by Will Schenk
- Using Thor and Ruby to build a CLI by Derik Ramirez
-
Supercharge Your Ruby CLI with Thor by Ian Hollander.
NB:option
was renamed tomethod_option
. - Introduction to Thor by Dhaivat Pandya
- Testing Thor Ruby Required Options by Stefanni Brasil