Published 2024-07-22.
Last modified 2024-07-31.
Time to read: 2 minutes.
posts
collection, categorized under JavaScript.
I like the tabs provided by
jSuites.tabs
because they work well, and they do not require jQuery
, so the generated tabs are lightweight.
However, the documentation is thin.
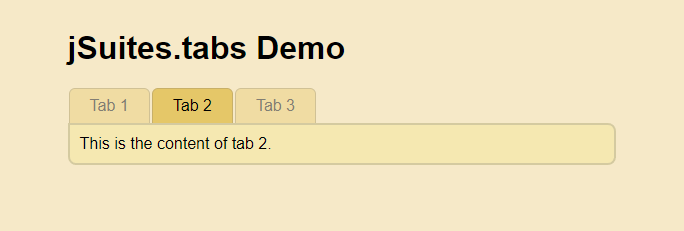
These are my notes.
URLs and URI Fragments
https://domain.com/jtabs_demo.html#tab2
Defining a Few Terms
I expect readers of this website will generally know what a Uniform Resource Locator (URL) is. Wikipedia has a good page if you would like a quick refresher.
A URI fragment
is delimited from the web page by an octothorpe (#), also known as a pound sign, also known as a hash mark.
For example, the URL http:/
has a URI fragment of tab2
.
Popular documentation in 2024 often refers to URI fragments as hashes, so we would normally say that
the hash value of the given URL is tab2
.
Two Uses For a URL Hash
- When serving a web page whose URL contains a hash, the user's web browser scans the web page, looking for an HTML element ID that matches the hash value. If a match is found, the web browser tries to scroll the web page up or down, so the element with the matched ID is as close to the top of the browser window as possible.
- A few lines of JavaScript can cause a URI fragment to activate a specific HTML tab among a set of tabs. I will show you how to do that using jSuites.tabs in this article.
Opening a Tab From a URL Hash
jSuites.tabs needs the following HTML structure to define a jtabs
instance for every element with class jtabs
.
The collection of jtabs is saved in the JavaScript variable, also called jtabs
:
<div class="jtabs"> <div id="jtabs-headers"> <div id="tab1">Tab 1</div> <div id="tab2">Tab 2</div> <div id="tab3">Tab 3</div> </div> <div id="jtabs-content"> <div> This is the content of tab 1. </div> <div> This is the content of tab 2. </div> <div> This is the content of tab 3. </div> </div> </div>
jtabs = jSuites.tabs(document.getElementsByClassName('jtabs')[0]);
The following 3 JavaScript incantations do the same thing in different ways:
they all use the URL hash to activate the second tab of the jtabs
instance,
labeled "Tab 2", in the following jSuites.tabs.
The following JavaScript statement invokes the open
method provided by the jtabs
instance and passes the value 1.
Because the first tab has index 0, the second tab has index 1.
The second tab is thus revealed and is made active.
jtabs.open(1); // origin 0
The following JavaScript statement also opens the tab with ID tab2
.
This JavaScript statement obtains the HTML element with ID jtabs2
and passes it to selectIndex
.
The second tab is then revealed and made active.
jtabs.selectIndex(document.getElementById("jtabs2"));
The third JavaScript incantation is the most interesting.
If a URI fragment (aka a hash) was specified, obtain the HTML element for that ID
and pass it to selectIndex
as before.
The second tab is then revealed and made active.
const desiredTab = window.location.hash.split('#')[1]; if (typeof desiredTab !== 'undefined') jtabs.selectIndex(document.getElementById(desiredTab));
jSuites.tabs Demo
I wrote this example to show how to style tabs and programmatically open them.
The following iframe
was embedded with URL jtabs_demo.html#tab2
,
which is why Tab 2 was active.
You can click on the tabs to verify that the page is live.
Here is the complete HTML for the above JTabs demo:
<html> <head> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/jsuites/dist/jsuites.min.css" type="text/css" /> <style> body { background-color: rgba(242, 215, 158, 0.55); font-family: "Helvetica Neue", Helvetica, Arial, sans-serif; font-size: 16px; margin-left: 10%; margin-top: 2em; width: 80%; } .jtabs-content { background-color: #F7E8B4; border: 2px solid rgba(102, 102, 102, 0.226); border-radius: 0 8px 8px 8px; padding: 10px; } .jtabs .jtabs-headers { div { border-color: rgba(102, 102, 102, 0.226); border-style: solid; border-width: 1px 1px 0 1px; border-top-left-radius: 6px; border-top-right-radius: 6px; margin-left: 6px; } div:not(.jtabs-selected) { background-color: rgba(216, 162, 1, 0.184); color: rgba(102, 102, 102, .8); } div.jtabs-selected { background-color: rgba(216, 162, 1, 0.479); color: black; } } </style> </head> <body> <h1>jSuites.tabs Demo</h1> <div class="jtabs"> <div id="jtabs-headers"> <div id="tab1">Tab 1</div> <div id="tab2">Tab 2</div> <div id="tab3">Tab 3</div> </div> <div id="jtabs-content"> <div> This is the content of tab 1. </div> <div> This is the content of tab 2. </div> <div> This is the content of tab 3. </div> </div> </div> </body> <script defer src="https://cdn.jsdelivr.net/npm/jsuites/dist/jsuites.min.js"></script> <script> document.addEventListener('DOMContentLoaded', function() { jtabs = jSuites.tabs(document.getElementsByClassName('jtabs')[0]); // The following two statements accomplish the same task: open tab 2 // jtabs.open(1); // origin 0 //jtabs.selectIndex(document.getElementById("tab2")); // The following opens tab2 if the URL ends with #tab2 const desiredTab = window.location.hash.split('#')[1]; if (typeof desiredTab !== 'undefined') jtabs.selectIndex(document.getElementById(desiredTab)); }); </script> </html>